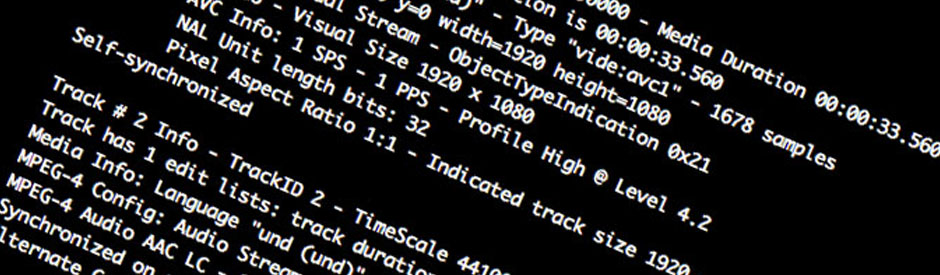
I’m working on a project where MP4 files are FTP’d over to a different server. This server’s job is to add a front and end bumper to the video clips automatically and host for user pickup. I needed to find a way to ensure that the source video had finished transferring and was complete before starting the process. I’ve found that MP4Box is up to the task.
For this I’m using a server with Ubuntu 24.04 LTS and PHP 8.4.
There’s a lot of hints out there that mplayer or ffmpeg could be used to detect the MP4 file integrity but I found that this didn’t integrate very well with PHP. After some digging I found a utility called MP4Box. First off we’ll need to install that.
Download and install gpac
sudo apt install gpac
Verify the installation.
which MP4Box
Now for the PHP part
Passing a file in to MP4Box’s -info function will give you a result really quickly and provide an error if the file is truncated.
Here’s an example response from MP4Box with an incomplete MP4 file…
user@ubuntu:~/mp4/incoming$ MP4Box -info 'broken.mp4'
[iso file] Incomplete box mdat - start 163840 size 32859
[iso file] Incomplete file while reading for dump - aborting parsing
MP4Box tells us that the file is truncated meaning that the transfer is either still in progress or has failed. We can now capture this error in PHP and only continue with a process if the file is fully there.
<?php
// Recurse through MP4 files within the incoming directory
$files = glob('./*.mp4');
// Loop through these files
foreach($files as $file) {
// Run MP4Box's -info function on the file
// You must include the '2>&1' here to see the output in PHP
$output = shell_exec( "MP4Box -info '$file' 2>&1" );
// If the output contains 'Incomplete' then the file is broken
if( strpos( $output, 'Incomplete' ) == true ){
echo $file . " is incomplete." . PHP_EOL;
}
else {
echo $file . " is complete." . PHP_EOL;
}
}
?>